A common user interface(UI) component is a content carousel or slider. It is often used to display images on a site and the user interacts with it by clicking on navigation arrows to change the content displayed.
In this post, we are going to see how we can build a custom slider that can display any type of html content; images, text and even videos. Let’s get started
What we are going to build
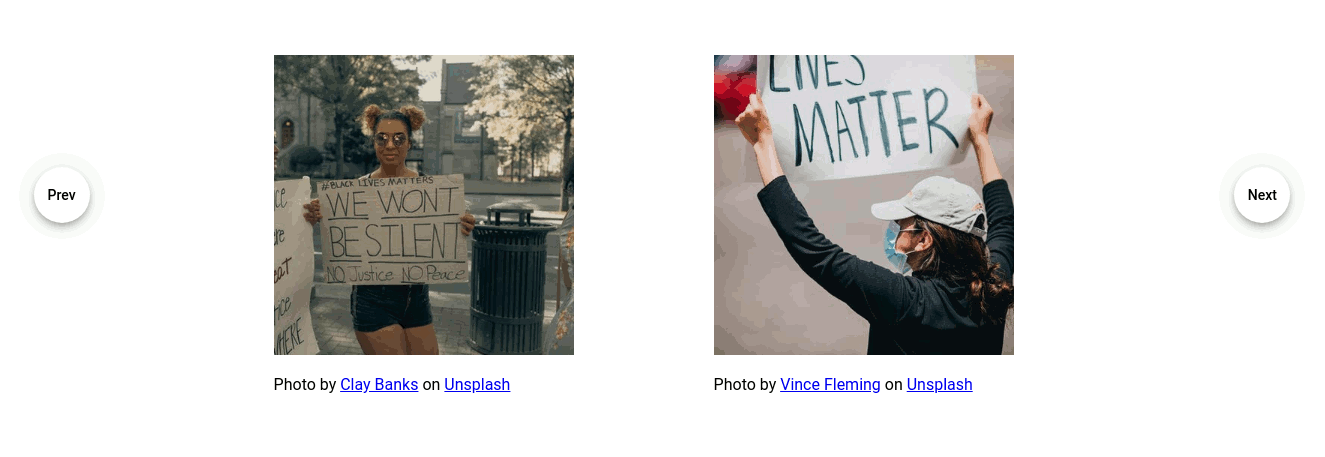
Prerequisite
- Angular
- Angular Material
I will be assuming you already have Angular installed and setup. If not, check Angular’s guide on getting started. You can also check out how to set up a local development environment for Angular.
Step 1: Add Angular Material
ng add @angular/material
This will add Angular Material, the Component Development Kit (CDK) and Angular Animation packages. All of which we will be using. It will also modify your package.json, adding some default values.
Step 2: Create the custom slider component
Create the component that will hold our custom slider
ng generate component custom-slider
In order to use the slider features provided by Angular material, our custom component needs to extend the CdkStepper component. We do that below
import { Component } from '@angular/core'; import { CdkStepper } from '@angular/cdk/stepper'; @Component({ selector: 'app-custom-slider', templateUrl: './custom-slider.component.html', styleUrls: ['./custom-slider.component.css'], providers: [{ provide: CdkStepper, useExisting: CustomSliderComponent }], }) export class CustomSliderComponent extends CdkStepper {}
First, we add CdkStepper to the providers array of the Component decorator. Specifying that it should use our CustomSliderComponent class.
Then we extend our class with the CdkStepper class.
At this point, our custom component will have access to all the methods and variables of CdkStepper.
Next step is to build the interface for our component
Step 3: Custom slider interface
<section class="container-fluid"> <div [style.display]="selected ? 'flex' : 'none'" class="row"> <!-- Content from the CdkStep is projected here --> <ng-container [ngTemplateOutlet]="selected.content"></ng-container> </div> <div class="custom-slider-navigation"> <button mat-fab aria-label="Previous" class="prev-btn" cdkStepperPrevious> <mat-icon>chevron_left</mat-icon> </button> <button mat-fab aria-label="Next" class="next-btn" cdkStepperNext> <mat-icon>chevron_right</mat-icon> </button> </div> </section>
Here, we just create a container to hold the html content, and add some buttons for navigation.
The key part above is
<ng-container [ngTemplateOutlet]="selected.content"></ng-container>
It allows the html contents provided to the custom slider to be displayed by the slider
Step 4: Add some styling
The CDK comes without any styling, so we can apply any style we want.
.content-slider-navigation { height: 100%; } .content-slider-navigation button { position: absolute; top: 40%; background-color: transparent; } .prev-btn { left: 0; } .next-btn { right: 0; }
Step 5: Using our custom slider
You can use the slider as shown below
<app-custom-slider> <cdk-step> <div class="image-holder"> <img src="https://source.unsplash.com/ug9umL90BvI/400x400" /> </div> <div class="image-holder"> <img src="https://source.unsplash.com/TCbkeGQM0DA/400x400" /> </div> </cdk-step> <cdk-step> <div class="image-holder"> <img src="https://source.unsplash.com/E77SjOPCE5Y/400x400" /> </div> <div class="image-holder"> <img src="https://source.unsplash.com/2Qb6XP67KjA/400x400" /> </div> </cdk-step> </app-custom-slider>
That is all. We should now be able to use our custom slider to display any html content as a slide. Because it is a component, we can reuse it throughout our application.
You can extend on this to add more styling and handle events based on the need of your project.
Feel free to reach out with any questions or comments.
Have any questions, want to share your thoughts or just say Hi? I’m always excited to connect! Follow me on Twitter or LinkedIn for more insights and discussions. If you’ve found this valuable, please consider sharing it on your social media. Your support through shares and follows means a lot to me!